Automatically debug memory leaks.
BLeak automatically locates, ranks, and diagnoses memory leaks in client-side web applications. Works on , , and .
Install today:
$ npm install -g bleak-detector
Builds on and requires MITMProxy 4
$ pip install mitmproxy==4.0.1
1. Write a configuration file.
The configuration file specifies a loop of screens (or "visual states") that BLeak will run through.
Code in the configuration file executes in the context of the web page, so you can interact with JavaScript running on the webpage.
Optional attributes specify one-time login and setup procedures to enter the loop.
2. Run BLeak.
Using the configuration file, BLeak runs your web application in a loop through the specified visual states and looks for memory leaks.
$ bleak run --config my.config.js \ --out bleak_output
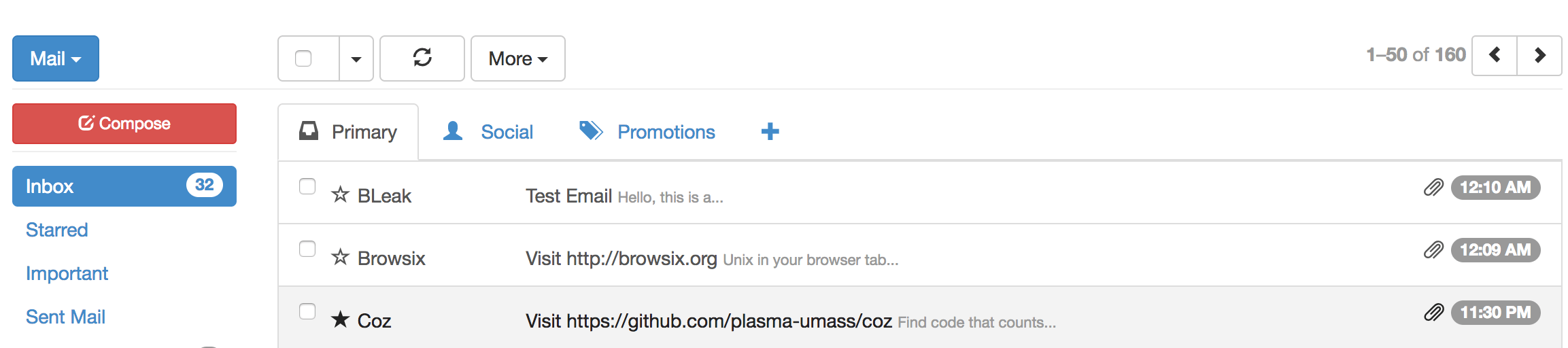
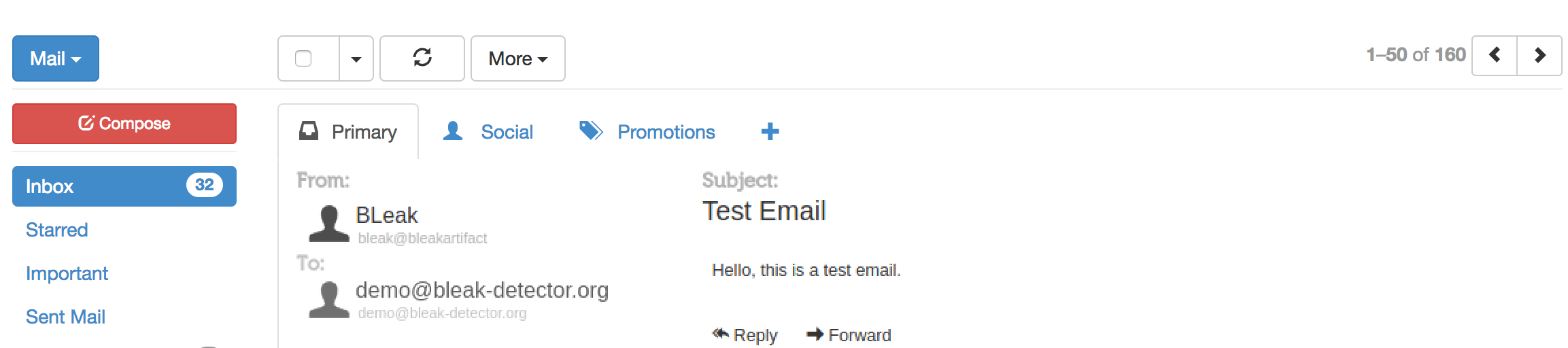
Specifically, BLeak looks for objects that are exhibiting sustained growth.
3. View results.
The BLeak viewer displays all of the information needed to debug each memory leak.
$ bleak viewer
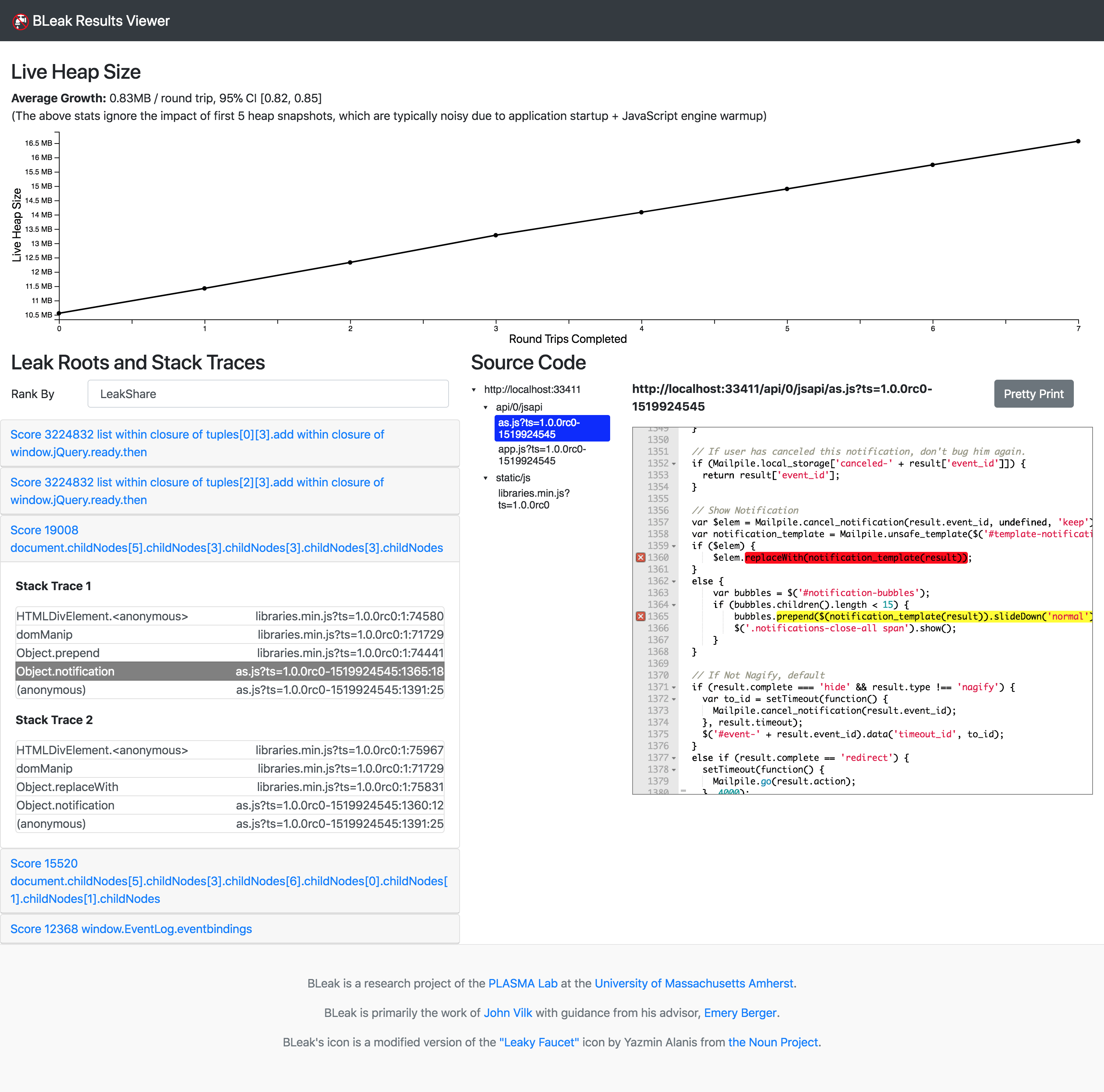
Specifically, the viewer displays the following information:
- The magnitude of heap growth over loop round trips
- The web application's source code, with problematic program statements highlighted
- For each leak:
- Human-readable heap paths at which the growing object is located
- A LeakShare score that represents the impact/importance of the memory leak
- Stack traces that point to the code responsible for growing the object
BLeak finds leaks in real web applications.
BLeak found and diagnosed over 50 memory leaks in dozens of libraries and applications, and can do so without access to unminified/unobfuscated source code. See our paper for a full listing.
Criteo OneTag
(Leak disclosed in email)